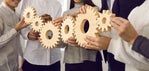
Orchestration and choreography in .NET microservices
Orchestration takes a centralized approach, and choreography a decentralized approach, to coordinating the interactions of microservices. Understand the differences.
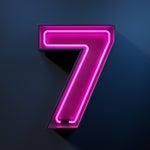
The best new features in ASP.NET Core 7
The latest version of Microsoft’s web application development framework brings excellent new capabilities to middleware, minimal API apps, and more. Here are the highlights.
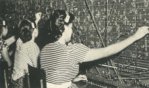
How to use implicit and explicit operators in C#
Take advantage of implicit and explicit operators to convert between user-defined types and improve the readability of your code.
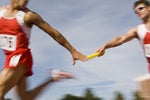
How to work with Action, Func, and Predicate delegates in C#
Learn how you can take advantage of delegates like Action, Func, and Predicate to facilitate callbacks and add flexibility to your code.
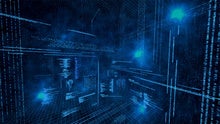
How to work with delegates in C#
Take advantage of delegates to promote flexibility and code reuse in your .NET applications and to implement event-driven programming with ease.
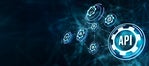
How to use OpenAPI in ASP.NET Core
Take advantage of the built-in support for OpenAPI in ASP.NET Core to automatically document your HTTP endpoints. Minimal APIs are supported too.

How to use the null object pattern in .NET
Take advantage of the null object pattern in .NET to eliminate the need for null checks and avoid runtime errors in your applications.
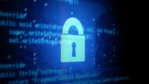
How to use symmetric and asymmetric encryption in C#
Learn how to protect the information handled by your .NET applications by encrypting and decrypting the data using either a single key or a public/private key pair.
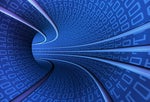
How to work with endpoint filters in ASP.NET Core 7
Take advantage of endpoint filters in ASP.NET Core 7 to modify request and response objects, short-circuit the request processing pipeline, or handle cross-cutting concerns for every request.
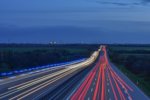
How to use BufferedStream and MemoryStream in C#
Take advantage of the stream classes in .NET 7 for faster reads and writes of the data in your .NET applications.

How to use EF Core query types in ASP.NET Core 7
Take advantage of query types in EF Core to query types that don’t have keys and to map to tables and views that lack an identity column.
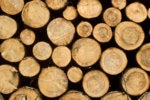
How to work with logging in EF Core 7
Learn how you can use Entity Framework Core to log data to the console, SQL Server, and other log targets when working with ASP.NET Core 7 applications.
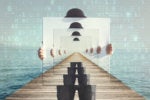
How to use the in, out, and ref keywords in .NET Core
Take advantage of the in, out, and ref keywords to pass parameters to your C# methods in .NET and make your code more readable and maintainable.
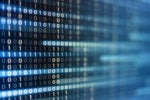
How to use BitArray in .NET 7
Take advantage of the BitArray class in .NET 7 to perform bitwise operations on your data for improved performance.
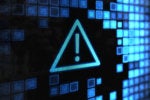
Use model validation in minimal APIs in ASP.NET Core 6
Take advantage of FluentValidation to validate your model classes when working with minimal APIs in ASP.NET Core 6.
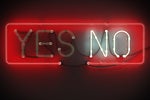
How to use cancellation tokens in ASP.NET Core 7
Take advantage of cancellation tokens in ASP.NET Core to allow long running operations to be cancelled gracefully and keep applications responsive.
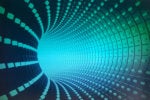
How to use route handler filters in minimal APIs in ASP.NET Core 7
Take advantage of minimal API filters in ASP.NET Core 7 to modify request and response objects or short-circuit the request processing pipeline.
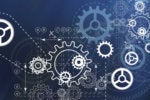
How to create a custom configuration provider in ASP.NET Core 6
ASP.NET Core configuration providers read configuration data from common sources such as JSON files and databases. But you can use other sources by creating custom configuration providers.
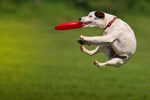
How to use EF Core as an in-memory database in ASP.NET Core 6
Entity Framework Core allows you to store and retrieve data to and from an in-memory database. It’s a quick and easy way to test your ASP.NET Core 6 web applications.
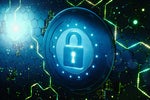
How to implement JWT authentication in ASP.NET Core 6
It’s easy to secure minimal API endpoints in ASP.NET Core 6 using JSON Web Tokens for authentication and authorization. Just follow these steps.